Frontend/JavaScript
[JavaScript] 코어 자바스크립트 7장 - 클래스
반응형
아래 책을 읽고 정리한 내용입니다.
http://www.yes24.com/Product/Goods/78586788
코어 자바스크립트 - YES24
자바스크립트의 근간을 이루는 핵심 이론들을 정확하게 이해하는 것을 목표로 합니다최근 웹 개발 진영은 빠르게 발전하고 있으며, 그 중심에는 자바스크립트가 있다고 해도 결코 과언이 아니
www.yes24.com
💡 자바스크립트의 클래스를 이해하기 위해서는 프로토타입의 이해가 우선되어야 한다.
자바스크립트에서의 클래스
- 자바스크립트는 프로토타입 기반 언어이므로 클래스의 개념이 존재하지 않는다.
- 그저 프로토타입을 클래스의 관점에서 접근해 상속 흉내를 것이다.
- 프로토타입 체이닝에 의한 참조를 상속으로 이해하는 것이다.
예시를 통해 자세히 알아보자.
- 아래
Array
와arr
를 클래스와 인스턴스 개념으로 보는 것이다. Array
의prototype
객체 내부 요소들이arr
의__proto__
로 상속된다고 보는 것이다. (스태틱 메서드)- 이때
Array
의prototype
을 제외한 메서드는arr
에 상속되지 않는다. (프로토타입 메서드)
const arr = new Array();
console.dir(Array);
console.dir(arr);
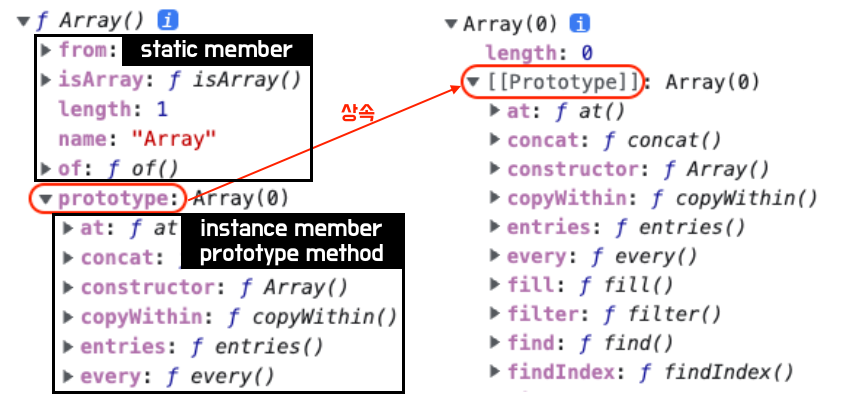
클래스 상속
자바스크립트에서 클래스 상속을 구현했다는 것은 결국 프로토타입 체이닝을 잘 연결한 것이다.
//Array의 prototype을 Grade에 연결
var Grade = function() {
var args = Array.prototype.slice.call(arguments);
for (var i = 0; i < args.length; i++) {
this[i] = args[i];
}
this.length = args.length;
};
Grade.prototype = [];
var g = new Grade(100, 80);
console.log(g); //Array { '0': 100, '1': 80, length: 2 }
다만, 위와 같이 프로타입을 직접 연결하는 것은 잘 구현된 것 같아도 여러 문제가 발생할 수 있기 때문에 구조적으로 불안정하다. 그리고 프로토타입 체인으로 상속을 흉내 내는 것은 꽤 복잡하다.
ES6 클래스 문법
ES6에서는 클래스와 상속을 간단하게 처리할 수 있는 클래스 문법이 도입되었다. ES5에서의 프로토타입과 ES6의 클래스 문법을 비교해 보자.
ES5의 생성자 함수와 프로토타입
var ES5 = function (name) {
this.name = name;
}
ES5.staticMethod = function(){
return this.name + ' staticMethod';
}
ES5.prototype.method = function(){
return this.name + ' method';
}
var es5Instance = new ES5('es5');
console.log(ES5.staticMethod()); //es5 staticMethod
console.log(es5Instance.method()); //es5 method
ES6의 클래스 문법
var ES6 = class {
constructor(name){
this.name = name;
}
static staticMethod (){
return this.name + ' staticMethod';
}
method(){
return this.name + ' method';
}
};
var es6Instance = new ES6('es6');
console.log(ES6.staticMethod()); //es6 staticMethod
console.log(es6Instance.method()); //es6 method
ES6의 클래스 상속
var Rectangle = class {
constructor(width, height){
this.width = width;
this.height = height;
}
getArea() {
return this.width * this.height;
}
};
var Square = class extends Rectangle {
constructor(width){
super(width, width);
}
};
var sq = new Square(5);
console.log(sq.getArea()); //25
반응형
'Frontend > JavaScript' 카테고리의 다른 글
[바닐라 자바스크립트로 SPA 만들기] 2. 비동기 처리 (0) | 2023.06.25 |
---|---|
[바닐라 자바스크립트로 SPA 만들기] 1. 컴포넌트 만들기 (0) | 2023.06.24 |
[JavaScript] 코어 자바스크립트 6장 - 프로토타입 (0) | 2023.06.07 |
[JavaScript] 코어 자바스크립트 5장 - 클로저 (0) | 2023.06.05 |
[JavaScript] 코어 자바스크립트 4장 - 콜백 함수 (0) | 2023.05.29 |